Publish and subscribe to notifications with AWS SNS
This guide explains how to integrate AWS SNS with a qibb flow using the function node and the official AWS SDK (https://www.npmjs.com/package/@aws-sdk/client-sns) imported as external module.
For details on how to configure external modules in a function node, please refer to How to use external modules in a function node.
The given code snippets are expecting the user to have secrets configured in Space Secrets (See Managing secrets in your space and accessing them from your flows ).
This guide is also available as a flow, which you directly import into the Flow Editor: flows_qibb_aws_sns.json
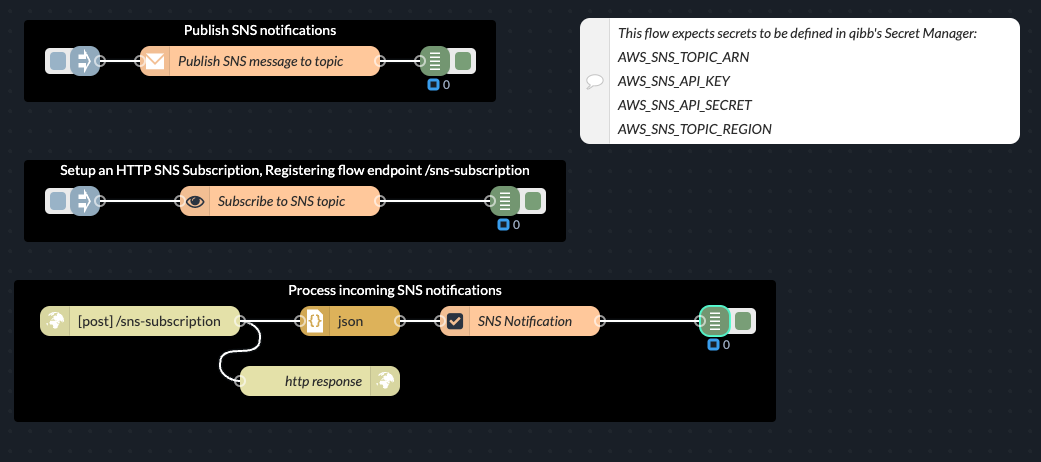
Using the function node and AWS SDK
The examples will use the function node to integrate with AWS SNS using the AWS SDK. As a prerequisite, according function nodes should have the @aws-sdk/client-sns
module installed and imported as awsSdkClientSns
.

Module in “Setup” tab of function node
In addition, the function node is expecting to have the following secrets stored in Space Secrets:
AWS_SNS_TOPIC_ARN
AWS_SNS_API_KEY
AWS_SNS_API_SECRET
AWS_SNS_TOPIC_REGION
Publishing SNS notifications
This example demonstrates how to publish a notification to an AWS SNS topic.

Publish SNS notification to a topic
Function Node Code Example for “Send SNS notification”
Function | Code |
---|---|
“On Start” Tab Handles configuration of client on whenever the flow starts or restarts. |
JS
|
“On Message” Tab Uses the client to send notification and passes response to output. |
JS
|
Subscribe and receive SNS notifications
This example shows how to subscribe for an AWS SNS topic, setting up an HTTPS subscription pointing to an endpoint of the flow. AWS SNS will call the given endpoint immediately to confirm the subscription. Once the subscription is confirmed, AWS SNS will call this endpoint every time there is a new notification for that topic, and the flow will process the messages accordingly.
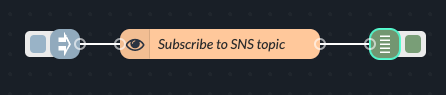
Subscribe to SNS topic
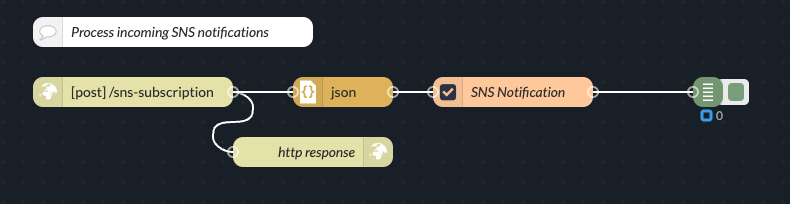
Process incoming SNS notifications
Function Node for “Subscribe to SNS topic”
Function | Code |
---|---|
“On Start” Tab Handles configuration of client on whenever the flow starts or restarts. |
JS
|
“On Message” Tab Uses the stored client to setup a subscription for the SNS topic. |
JS
|
Function Node for “Processing SNS Notification”
Function | Code |
---|---|
“On Start” Tab | No code required. |
“On Message” Tab Checks the type of the incoming message. If it has the type SubscriptionConfirmation, makes an HTTP request back to AWS SNS to confirm subscription. If it has the type Notification, it parses and passes the message to the node output. |
JS
|